SpringBoot使用矩阵传参
一、介绍
在平时,我们在进行请求接口时,我们一个请求url
的样子往往是下面这样子的
1
| http://localhost:8080/user/get?name=半月&age=18
|
对于上面的请求url
,我们只需要使用@RequestParam
注解就可以将其获取,十分简单。
那么,对于下面的这个矩阵传参的url
,我们该如何进行获取呢?
1 2 3
| http://localhost:8080/user/get;name=半月;age=18
http://localhost:8080/user/delete;id=11,12,13;status=1
|
这时候,我们就该使用到@MatrixVariable
这个注解了,具体使用如下。
二、使用
1)基本使用
在springBoot
中,默认是去掉了url
分号后的内容。如此一来,我们在使用矩阵传参时,需要对其进行开启。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.banmoon.test.config;
import org.springframework.context.annotation.Configuration; import org.springframework.web.servlet.config.annotation.PathMatchConfigurer; import org.springframework.web.servlet.config.annotation.WebMvcConfigurer; import org.springframework.web.util.UrlPathHelper;
@Configuration public class MyWebMvcConfig implements WebMvcConfigurer {
@Override public void configurePathMatch(PathMatchConfigurer configurer) { UrlPathHelper urlPathHelper = new UrlPathHelper(); urlPathHelper.setRemoveSemicolonContent(false); configurer.setUrlPathHelper(urlPathHelper); } }
|
或者可以这样写,效果都是一样的,熟悉@bean
配置的大家都知道。推荐使用上面这种,简单明了
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.banmoon.test.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.web.servlet.config.annotation.PathMatchConfigurer; import org.springframework.web.servlet.config.annotation.WebMvcConfigurer; import org.springframework.web.util.UrlPathHelper;
@Configuration public class MyBeanConfig {
@Bean public WebMvcConfigurer webMvcConfigurer(){ WebMvcConfigurer webMvcConfigurer = new WebMvcConfigurer() { @Override public void configurePathMatch(PathMatchConfigurer configurer) { UrlPathHelper urlPathHelper = new UrlPathHelper(); urlPathHelper.setRemoveSemicolonContent(false); configurer.setUrlPathHelper(urlPathHelper); } }; return webMvcConfigurer; } }
|
对于下面这两个url
请求,我们可以这样写接口
1 2 3
| http://localhost:8080/user/get/get;name=半月;age=18
http://localhost:8080/user/delete/delete;name=半月;age=18,19,20
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.banmoon.test.controller;
import com.banmoon.test.dto.ResultData; import org.springframework.web.bind.annotation.*;
import java.util.HashMap; import java.util.Map;
@RestController @RequestMapping("user") public class UserMatrixVariableController {
@GetMapping("/get/{path}") public ResultData get(@MatrixVariable String name, @MatrixVariable String age, @PathVariable String path){ Map<String, Object> map = new HashMap<>(); map.put("name", name); map.put("age", age); map.put("path", path); return ResultData.success(map); }
}
|
请求查看结果
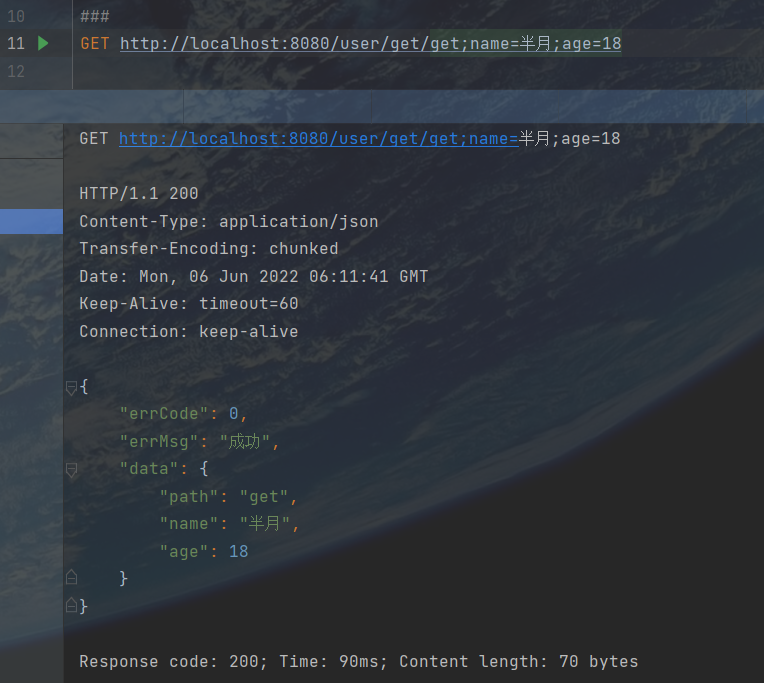
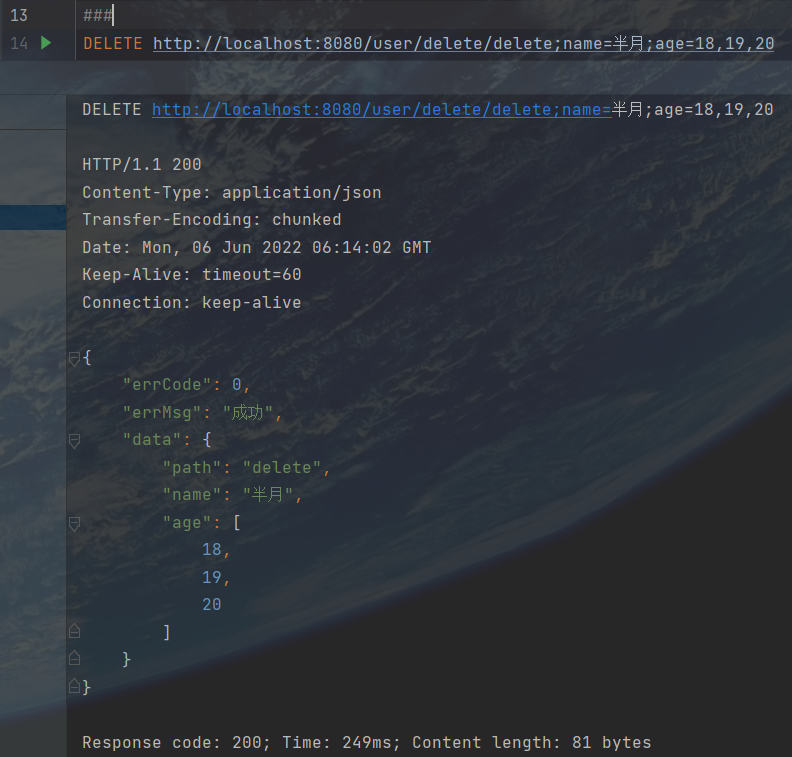
2)其他
另外一种请求url
,看着很乱
1
| http://localhost:8080/user/setUserPost/101;status=1/post/111;status=1
|
接口是这样的
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package com.banmoon.test.controller;
import com.banmoon.test.dto.ResultData; import org.springframework.web.bind.annotation.*;
import java.util.HashMap; import java.util.Map;
@RestController @RequestMapping("/user") public class UserMatrixVariableController {
@GetMapping("/setUserPost/{userId}/post/{postId}") public ResultData setUserPost(@PathVariable Integer userId, @PathVariable Integer postId, @MatrixVariable(value = "status", pathVar = "userId") Integer userStatus, @MatrixVariable(value = "status", pathVar = "postId") Integer postStatus){ Map<String, Object> map = new HashMap<>(); map.put("userId", userId); map.put("postId", postId); map.put("userStatus", userStatus); map.put("postStatus", postStatus); return ResultData.success(); }
}
|
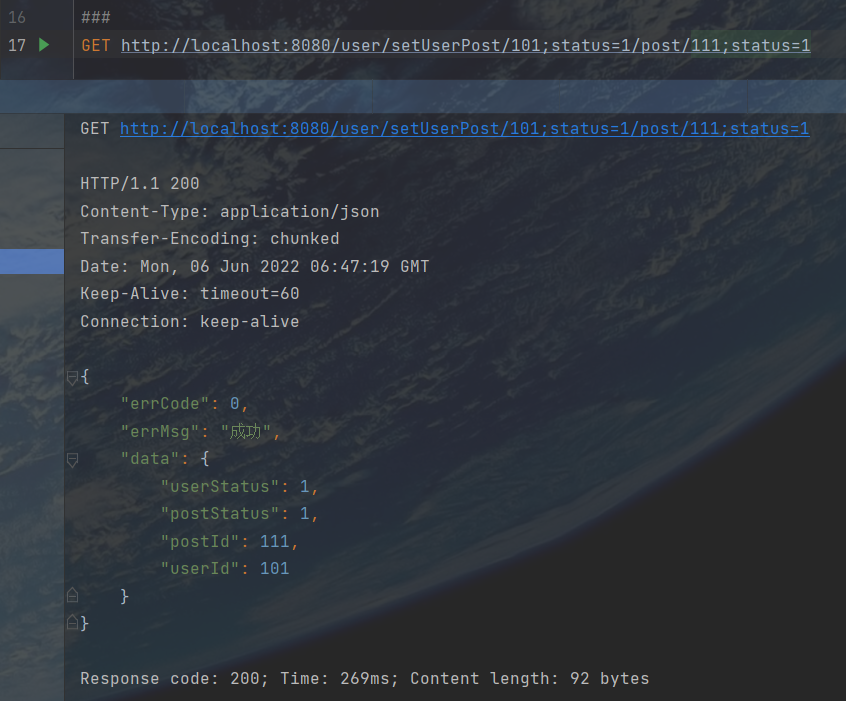
三、最后
在处理完矩阵传参后,我就知道这玩意有多么不受待见了,我也搞不懂会有什么样的业务场景去使用这种传参模式。
好吧,可以不用,但不能不知道。
我是半月,祝你幸福!!!