SpringBoot实现统一响应提示国际化
一、介绍
统一响应大家都见识过,只需要添加上@RestControllerAdvice
后进行处理即可
简单示例如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.banmoon.test.core.handler;
import com.banmoon.test.obj.dto.ResultData; import lombok.extern.slf4j.Slf4j; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.RestControllerAdvice;
@Slf4j @RestControllerAdvice public class ControllerHandler { @ExceptionHandler(Exception.class) public ResultData exceptionHandler(Exception exception){ log.error("统一异常", exception); return ResultData.fail("服务器繁忙,请稍后再试!"); } }
|
这里的服务器繁忙,请稍后再试!,仅仅只有中文,能不能实现国际化的语言呢
本文将介绍使用i18n
,来完成国际化语言的使用。
i18n
是什么,全称internationalization
,取首字母,尾字母,18
代表中间的十八个字母。
在spring
中使用i18n
来完成国际化
二、代码
在spring
中,有这么一个接口MessageSource
,它是专门用来解决国际化问题的。
其中有这么一个实现类ResourceBundleMessageSource
,从配置文件中读取国际化的语言,进行展示
首先,我们得准备好三份配置文件,分别如下
1 2 3
| default=Server busy, please try again later! 1000=The record already exists in the database 2000=Uploading file exception
|
1 2 3
| default=服务器繁忙,请稍后再试! 1000=数据库中已存在该记录 2000=上传文件异常
|
1 2 3
| default=服務器繁忙,請稍後再試! 1000=數據庫中已存在該記錄 2000=上傳文件異常
|
注意命名格式,这很重要,这样即可名称_[语言]_[国家].properties
准备好配置文件后,我将进行加载,创建出一个ResourceBundleMessageSource
的bean
,加载上面的配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.banmoon.test.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.support.ResourceBundleMessageSource;
@Configuration public class MessageSourceConfig {
@Bean public ResourceBundleMessageSource messageSource() { ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource(); messageSource.setBasenames("i18n/exceptionMessage"); return messageSource; }
}
|
如此,国际化的语言就配置完毕,接下来就是读取了
再写一个工具类用来读取
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| package com.banmoon.test.utils;
import cn.hutool.core.util.ArrayUtil; import cn.hutool.extra.spring.SpringUtil; import org.springframework.context.MessageSource;
import java.util.Locale;
import static java.util.Locale.CHINA;
public class MessageSourceUtil {
public static final MessageSource messageSource;
public static final String i18nStr;
public static final Locale locale;
static { messageSource = SpringUtil.getBean("messageSource"); i18nStr = SpringUtil.getProperty("i18n"); String[] arr = i18nStr.split("_"); locale = ArrayUtil.isNotEmpty(arr) ? new Locale(arr[0], arr[1]) : CHINA; }
public static String getMessage(Integer code, Object... objs) { return messageSource.getMessage(String.valueOf(code), objs, locale); }
public static String getMessage(String code, Object... objs) { return messageSource.getMessage(code, objs, locale); }
}
|
指定使用哪种语言环境
这个看大家以后国际化的定制,如何确定当前的语言环境
本文是由配置文件进行配置的,读取并生成Locale
对象
最后,就是统一异常捕获的相关类了
自定义异常BanmoonException.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.banmoon.test.core.exception;
import com.banmoon.test.utils.MessageSourceUtil; import lombok.Data; import lombok.NoArgsConstructor;
@Data @NoArgsConstructor public class BanmoonException extends RuntimeException {
private Integer code;
private String msg;
public BanmoonException(Integer code) { this(code, MessageSourceUtil.getMessage(code)); }
public BanmoonException(Integer code, String msg) { super(msg); this.code = code; this.msg = msg; } }
|
统一异常拦截类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package com.banmoon.test.core.handler;
import com.banmoon.test.core.exception.BanmoonException; import com.banmoon.test.obj.dto.ResultData; import com.banmoon.test.utils.MessageSourceUtil; import lombok.extern.slf4j.Slf4j; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.RestControllerAdvice;
@Slf4j @RestControllerAdvice public class ControllerHandler {
@ExceptionHandler(Exception.class) public ResultData<?> exceptionHandler(Exception exception){ log.error("统一异常", exception); return ResultData.fail(MessageSourceUtil.getMessage("default")); }
@ExceptionHandler(BanmoonException.class) public ResultData<?> BanmoonExceptionHandler(BanmoonException exception){ log.error("自定义异常", exception); Integer code = exception.getCode(); return ResultData.fail(code, MessageSourceUtil.getMessage(code)); } }
|
三、测试
测试就使用一个接口进行测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package com.banmoon.test.controller;
import com.banmoon.test.core.exception.BanmoonException; import com.banmoon.test.obj.dto.ResultData; import io.swagger.annotations.Api; import lombok.extern.slf4j.Slf4j; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController;
import java.util.Objects;
@Slf4j @Api(tags = "国际化测试") @RestController public class MessageSourceController {
@GetMapping("i18n") public ResultData<String> i18n(@RequestParam(required = false) Integer code) { if (Objects.nonNull(code)) { throw new BanmoonException(code); } else { int i = 1 / 0; } return new ResultData<>(); }
}
|
启动服务,请求进行测试
请求 |
配置文件 |
结果 |
 |
i18n: en_US |
 |
 |
i18n: en_US |
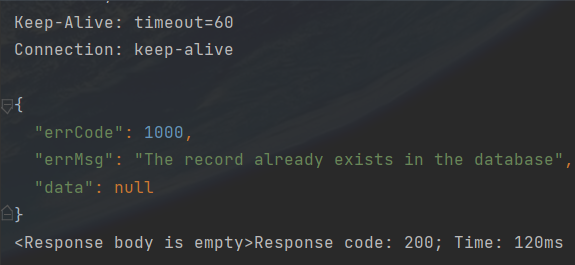 |
 |
i18n: zh_TW |
 |
 |
i18n: zh_TW |
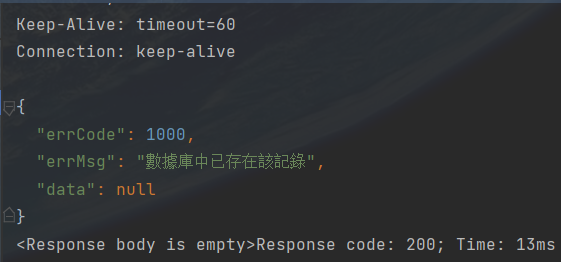 |
四、最后
在使用ResourceBundleMessageSource.java
实现类时,也可以关注其它的一些实现类,其中
我是半月,你我一同共勉!!!